From simple line charts to complex hierarchical tree maps, the Google Chart gallery provides a large number of ready-to-use chart types:
- Scatter Chart
- Line Chart
- Bar / Column Chart
- Area Chart
- Pie Chart
- Donut Chart
- Org Chart
- Map / Geo Chart
Bar Chart
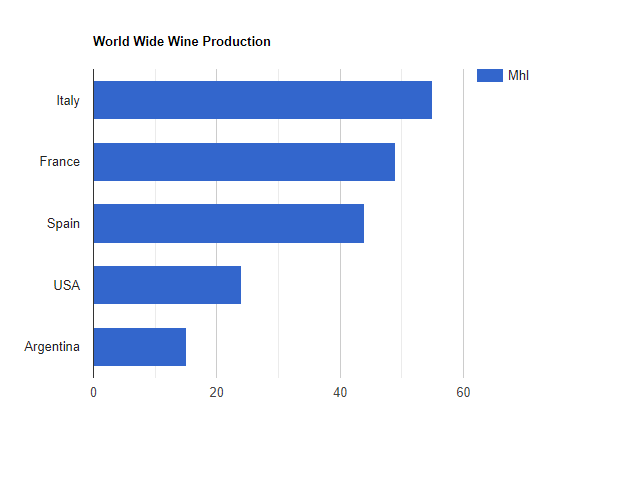
<!DOCTYPE html>
<html>
<script src="https://www.gstatic.com/charts/loader.js"></script>
<body>
<div id="myChart" style="width:100%; max-width:600px; height:500px;"></div>
<script>
google.charts.load('current', {'packages':['corechart']});
google.charts.setOnLoadCallback(drawChart);
function drawChart() {
// Set Data
const data = google.visualization.arrayToDataTable([
['Contry', 'Mhl'],
['Italy',55],
['France',49],
['Spain',44],
['USA',24],
['Argentina',15]
]);
// Set Options
const options = {
title:'World Wide Wine Production'
};
// Draw
const chart = new google.visualization.BarChart(document.getElementById('myChart'));
chart.draw(data, options);
}
</script>
</body>
</html>
Pie Chart
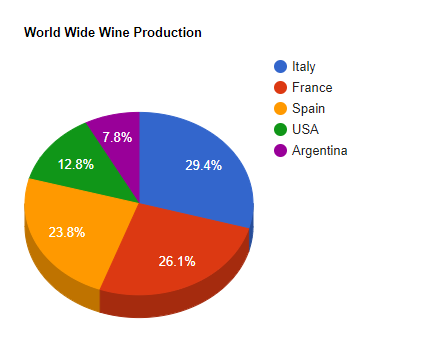
<!DOCTYPE html>
<html>
<script src="https://www.gstatic.com/charts/loader.js"></script>
<body>
<div
id="myChart" style="width:100%; max-width:600px; height:500px;">
</div>
<script>
google.charts.load('current', {'packages':['corechart']});
google.charts.setOnLoadCallback(drawChart);
function drawChart() {
// Set Data
const data = google.visualization.arrayToDataTable([
['Contry', 'Mhl'],
['Italy',54.8],
['France',48.6],
['Spain',44.4],
['USA',23.9],
['Argentina',14.5]
]);
// Set Options
const options = {
title:'World Wide Wine Production',
is3D:true
};
// Draw
const chart = new google.visualization.PieChart(document.getElementById('myChart'));
chart.draw(data, options);
}
</script>
</body>
</html>